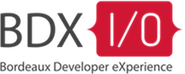
@binout https://github.com/binout
FEIGN + CREST = REST CLI
librairie pour faire un client http
librairie pour faire une Command Line Interface
Je veux faire un outil en ligne de commande pour gérer ma Dropbox
<dependency>
<groupId>com.netflix.feign</groupId>
<artifactId>feign-core</artifactId>
<version>${feign.version}</version>
</dependency>
public interface Dropbox {
@RequestLine("POST /files/list_folder")
@Headers("Content-Type: application/json")
FolderList listFolder(Path path);
@RequestLine("POST /files/create_folder")
@Headers("Content-Type: application/json")
void createFolder(Path path);
@RequestLine("POST /users/get_current_account")
Account currentAccount();
static Dropbox api() {
return Feign.builder()
.encoder(new GsonEncoder())
.decoder(new GsonDecoder())
.requestInterceptor(r -> r.header("Authorization", "Bearer " + apiKey()))
.target(Dropbox.class, "https://api.dropboxapi.com/2");
}
public static void main(String[] args) {
Dropbox.api()
.listFolder(new Path(""))
.stream()
.map(Folder::getName)
.forEach(System.out::println);
}
/Photos
/Public
/Documents
<dependency>
<groupId>org.tomitribe</groupId>
<artifactId>tomitribe-crest-api</artifactId>
<version>${tomitribe.version}</version>
</dependency>
<dependency>
<groupId>org.tomitribe</groupId>
<artifactId>tomitribe-crest</artifactId>
<version>${tomitribe.version}</version>
<scope>runtime</scope>
</dependency>
@Command
public String whoami() {
return API.currentAccount().getName().getDisplayName();
}
@Command
public void mkdir(Path path) {
API.createFolder(path);
}
@Command
public String ls(@Option("path") @Default("") Path path) {
return API.listFolder(path).stream()
.map(Folder::getName)
.collect(joining(lineSeparator()));
}
@Options
est
un paramètre falculatif de la commande@Default
permet de donner une valeur par défaut d’une option$ java -jar dropbox-cli.jar mkdir
Missing argument: Path...
Usage: mkdir Path
Crest fournit une classe Main par défaut : org.tomitribe.crest.Main
|
help
, exit
, clear
…|
<dependency>
<groupId>org.tomitribe</groupId>
<artifactId>tomitribe-crest-cli</artifactId>
<version>${tomitribe.version}</version>
</dependency>
public static void main(String[] args) throws Exception {
new CrestCli().run(args);
}
$ java -jar dropbox-cli.jar
benoit@1.8.0 $ help
Commands:
clear
exit
help
ls
mkdir
whoami
benoit@1.8.0 $ whoami
Benoit Prioux
Très peu de code pour faire un client http en ligne de commande :
Feign
(+ les POJOs pour le mapping Json)CREST
Ajouter une nouvelle commande :
Feign
Crest
Et si on codait ensemble la génération de lien temporaire ?
POST /sharing/create_shared_link_with_settings
Content-Type: application/json
{
"path": "...",
"settings": {}
}
/